Analysis
Purpose of software
An analyst will meet with the client when developing the software to discuss the purpose of the software.
The purpose of a piece of software is often a description of what the software will be used for.
Functional requirements
For National 5, the Analysis stage covers just functional requirements in the Software Design and Development unit (contrast this to the Web Design and Development unit where you cover user requirements also).
Functional requirements will specify the input, processes and outputs of a program.
Example
A program is required to store 3 test marks for a pupil. The test marks are percentages. The program should then calculate the average.
Functional requirements
Inputs | Processes | Outputs |
---|---|---|
3 test marks | Validate the inputs are between 0 and 100 inclusive | Average |
Calculate the total of all marks | ||
Calculate the average of the marks |
Assumptions
You may also need to make your own assumptions based on the software that could take account of the hardware or software needed to run the software (for example if the program was written in Python then the client's computer will need a Python interpreter to run it), compatibility concerns, whether an internet connection is required or not and the digital competence of the target group. These are just examples - there are plenty more things you may need to think about.
Design
Flowcharts
A flowchart is a graphical representation of a process or algorithm. It uses different shapes to represent different types of steps or processes and arrows to indicate the flow or sequence of these steps.
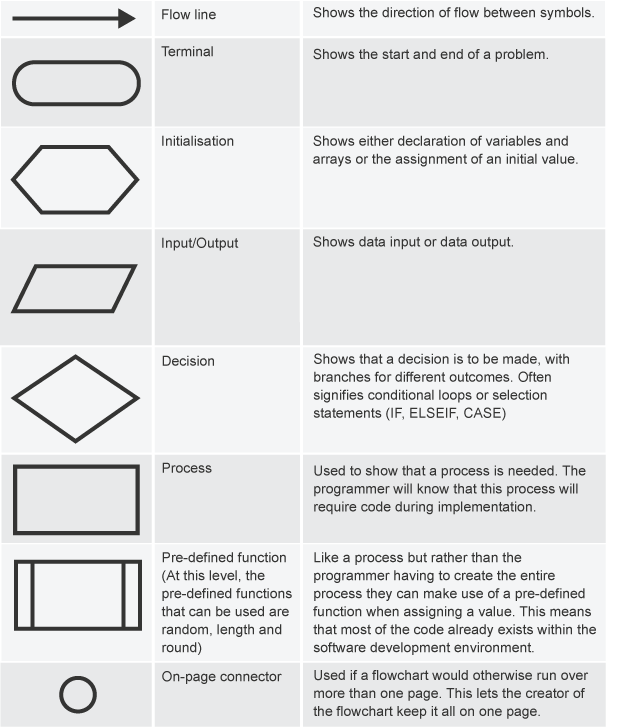
Symbols
- Oval (Start/End) Represents the start or end of a process.
- Rectangle (Process) Represents a task or operation.
- Diamond (Decision) Represents a decision point, usually with a yes/no answer.
- Parallelogram (Input/Output) Represents input or output operations.
- Arrow (Flow Direction) Indicates the flow or sequence of steps.
Flowcharts typically read from top to bottom and left to right. Arrows show the direction of flow.
Symbol meanings
- Start with an oval for the start point.
- Use rectangles for processes.
- Use diamonds for decision points.
- Use parallelograms for input/output.
- Connect the shapes with arrows to show the flow.
Decisions (selection and loops)
- Decision diamonds have conditions written inside.
- Arrows leaving the decision diamond represent possible outcomes based on the condition.
Loops and Repetition
- Use a rectangle for the process to be repeated.
- Use a diamond to represent the decision point for the loop.
- Connect the diamond back to the start of the loop process.
Connector Symbols
Use on-page connectors (small circles) to indicate where the flow continues on another part of the page.
Structure diagrams
Structure diagrams are another visual technique. They work using shapes in the same way that flowcharts do, but they use different shapes.
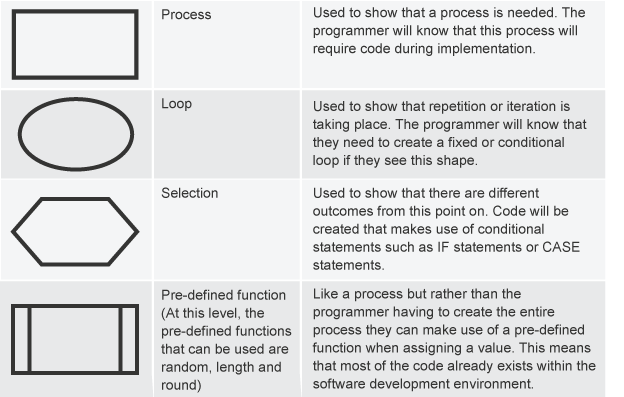
When reading a structure diagram you work from the top to the bottom of it first if a level contains more than one branch, read from left to right before moving back up the way.
Pseudocode
Pseudocode is another design technique like flowcharts and structure diagrams, but it is a written design notation instead of a visual technique.
Pseudocode is a middle ground between formal code syntaxes like Python and English.
Pseudocode uses indentation to make structures like selection (if
) and loops (for
and while
) more obvious.
To write pseudocode:
- Write down the main steps of the algorithm
- Refine the main steps where you can
- You do not always have to define variables, but you must remember to assign them!
- Use indentation for structures like selection and loops
- Pseudocode is not the same as a programming language. Do not muddle the two up
Implementation: Data Types and Structures
Implementation is the third step of the waterfall model.
In the implementation stage, the program is developed.
Data types
For National 5, you need to know about 5 data types:
Data type | Description | Example |
---|---|---|
Integer | A whole number | 4 |
Real | Stores a decimal number | 3.14 |
Boolean | True or False | True |
String | Alphanumeric values - a combination of characters and numbers as well as symbols. Integers or reals stored in strings have no value and cannot be used in calculations. We can however cast them (int() does this). |
"Hello world" |
Character | A character is just a single character. Python doesn't distinguish between characters and strings but you need to know what they are. | "A" |
Arrays
An array allows a programmer to store multiple pieces of related data in a single variable (like a list).
Below is an example of an array with five spaces (elements) in it which is stored in the variable marks
.
Arrays are accessed by their element position, known as their index or subscript. Remember that arrays start at position 0.
marks[1] = 55
In Python we can write this using the following code:
marks = [0] * 5 marks[0] = 31 marks[1] = 55
Implementation: Computational Constructs
Variables
Variables are parts of the computer's memory (RAM) that are allocated for storing data while the program is running.
A variable is given an identifier (a name), a data type (string etc.) and more often than not an initial value.
This can be written in Python using the following code:
forename = "John"
Mathematics
Programming languages can easily handle mathematical evaluation between multiple expressions.
This can written in Python using the following code:
value = 5 result = 7 + value
String concatenation
String concatenation is all about joining two or more strings together.
In SQA Reference Language you'll often see the &
symbol to represent concatenation:
Conditional statements
Operator | Python operator | Explanation | Example |
---|---|---|---|
< |
< |
Less than | age < 18 |
> |
> |
More than | age > 18 |
≤ |
<= |
Less than or equal to (sometimes shown as <=) | age ≤ 18 |
≥ |
>= |
More than or equal to (sometimes shown as >=) | age ≥ 18 |
= |
== |
Equality | age = 18 |
≠ |
!= |
Inequality/Not equal to | age ≠ 18 |
The previous are known as logical comparators.
The next table contains a list of all the logical operators.
Operator | Python operator | The result is true when... | Example of true |
---|---|---|---|
AND | and |
Both parts of the condition are true. | True and True |
OR | or |
At least one part of the condition is true. | True or False |
NOT | not |
Flips the value. So if true becomes false and false becomes true | not(False) |
Selection
A computer program is designed to be able to make decisions based on some input. To do this, we use selection. A selection statement is also known as an if statement.
DECLARE parkingSpacesUsed AS INTEGER INITIALLY 0
RECEIVE parkingSpacesUsed FROM KEYBOARD
IF parkingSpacesUsed > totalParkingSpaces THEN
SEND "You have entered a number of spaces used more that is more than the number of spaces available" TO DISPLAY
SET parkingSpacesUsed TO totalParkingSpaces
END IF
totalParkingSpaces = 78 parkingSpacesUsed = int(input("Please enter how many spaces are already used.")) if parkingSpacesUsed > totalParkingSpaces: print("You have entered a number of spaces used more that is more than the number of spaces available") parkingSpacesUsed = totalParkingSpaces
SEND "You are too young to drive" TO DISPLAY
ELSE
SEND "You are old enough to drive" TO DISPLAY
END IF
Note the use of indentation here that assists in showing the selection structure.
age = 18 if age < 17: print("You are too young to drive") else print("You are old enough to drive")
Fixed loops
A fixed loop is used to repeat the same thing a set number of times. If you wanted to print something ten times on to the screen you could use the following code:
SEND "Hello world!" TO DISPLAY
END REPEAT
for i in range(10): print("Hello world!")
The next example uses a loop variable to keep track of how many times the loop has run:
SEND counter TO DISPLAY
END FOR
for counter in range(0, 30): print(counter)
Conditional loops
Conditional loops repeat something until a condition has been met/satisfied (becomes true).
Here is an example of a conditional loop that acts as input validation as well:
RECEIVE password FROM (STRING) KEYBOARD
WHILE password != "password101" DO
SEND "Incorrect password" TO DISPLAY
RECEIVE password FROM (STRING) KEYBOARD
END WHILE
Below is the same algorithm in Python:
password = input("Input your password") while password != "password101": print("Incorrect password") password = input("Input your password")
Predefined functions
Functions are pieces of code, like print
that carry out a process (we need not know how it is carried out, only that it does it). Functions always return at least one value back which we can then use in our programs, for example by storing them in variables. Predefined functions are functions that are built-in to a language runtime such as Python.
For National 5, you need to know about 3 predefined functions:
- Length
- Random
- Round
You need to know how to use these functions as well as what the parameters mean.
Length
The length predefined function is used to get the length of a string or an array. The following will set a variable called strLength
to the length of a string variable containing the words
SET strLength TO LENGTH (str)
SEND strLength TO DISPLAY
In Python, this would look like:
str = "This is a string" strLength = len(str) print(strLength)
len
predefined function above, the parameter is str
.Random
The random function is used to get a random number between two numbers. It is used in SQA Reference Language as shown below:
SEND number TO DISPLAY
This will pick a number between 1 and 100. 1 is known as the low number and 100 is known as the high number.
To write this in Python you could write:
import random number = random.randint(0, 100) print(number)
Round
The round predefined function will round a real number to a certain number of decimal places. It takes two parameters, the first is a number to be rounded and the second is the number of decimal places to round to. In SQA Reference Language it looks like the following:
SET rounded TO ROUND(pi, 2)
SEND rounded TO DISPLAY
And the same code in Python:
pi = 3.14159 rounded = round(pi, 2) print(rounded)
Implementation: Algorithm Specification
Standard algorithms are algorithms you need to know how to produce in both pseudocode and Python.
Input validation
Input validation is used to validate that an input is within a valid range.
The following example expects the user to type in a number between 0 and 100, inclusive (this means it includes 0 and 100 as acceptable values).
SEND "Enter a number between 0 and 100" TO DISPLAY
RECEIVE numb FROM (INTEGER) KEYBOARD
WHILE numb < 0 OR numb > 100 DO
SEND "The number must be between 0 and 100 inclusive" TO DISPLAY
SEND "Enter a number between 0 and 100" TO DISPLAY
RECEIVE numb FROM (INTEGER) KEYBOARD
END WHILE
Below is the same algorithm in Python:
numb = int(input("Enter a number between 0 and 100")) while numb < 0 or numb > 100: print("The number must be between 0 and 100 inclusive") password = input("Enter a number between 0 and 100")
Running total
A running total algorithm will add a range of values together and provide a total. This total could be used to get an average of a list or set of numbers.
The following example adds all numbers defined in an array of numbers.
DECLARE marks INITIALLY [43, 12, 87]
FOR EACH mark FROM marks DO
SET total TO total + mark
END FOR EACH
Below is the same running total algorithm in Python:
total = 0 marks = [43, 12, 87] for i in range (0, len(marks)) total = total + marks[i]
Alternatively, a running total algorithm could be used to ask the user for a series of numbers and each number given is added to a total.
The following demonstrates this:
FOR counter FROM 0 TO 10 DO
SEND "Enter a mark" TO DISPLAY
RECEIVE mark FROM (INTEGER) KEYBOARD
SET total TO total + mark
END FOR
Below is the same running total algorithm in Python:
total = 0 for i in range (0, len(marks)) marks = int(input("Enter a mark")) total = total + mark
Testing
Testing of a program involves testing it with different test data. There are three types of test data, normal, extreme and exceptional.
Assume we have a program that is expected to take test score percentages (0 - 100), then the following table contains test data for this program:
Test data | What the program should do | Test data type |
---|---|---|
53 | Data is within the range of accepted values and the program should accept it | Normal |
98 | Data is within the range of accepted values and the program should accept it | Normal |
0 | Data is at the lower limit of the range of accepted data | Extreme |
100 | Data is at the upper limit of the range of accepted data | Extreme |
-1 | Data should not be accepted by the program | Exceptional |
101 | Data should not be accepted by the program | Exceptional |
"Test" | Data should not be accepted by the program | Exceptional |
Types of errors
We all make mistakes from time to time!
There are three types of errors in computer programs you need to know about for this course:
- Syntax
- Logic
- Runtime (execution)
Syntax errors
Syntax errors occur when there are grammatical or spelling mistakes in the code. A spelling mistake will cause the compiler to find a token in the wrong place and a grammatical error will cause the compiler to find a token it does not understand the meaning of.
Both types of these syntax errors will prevent the conversion of the code to something a computer can understand and will cause both an interpreter and compiler to crash.
Logic errors
Logic errors occur when the code produces an unexpected result.
In terms of logic errors, this occurs because of incorrect use of and
,
or
and/or not
operators in the code. This could mean
having or
where it was meant to have and
, for example
in a range check:
number = int(input("Please enter a number")) if number < 30 And number > 50) print("The number is within the range")
As you can see from the above code, this will never produce the correct result, because a number cannot be both less than 30 and greater than 50 at the same time.
Logic errors are more difficult to find than syntax or runtime errors since they do not cause the program to crash, but using breakpoints can help you find them.
Runtime errors
A runtime error or execution error occurs while the program is running. This is when a program is asked to do something it cannot do. This results in the program crashing. Common examples of this are attempting to access an array element that does not exist, dividing by 0, trying to access a file that does not exist, and in most programming languages (though not necessarily in Python), using a variable before it has been declared (this is, however, often flagged up by the compiler).
Evaluation
At National 5, you need to be able to evaluate whether a program is fit for purpose and whether or not it uses efficient constructs (note this is not the same as evaluating the efficiency of code).
Fitness for purpose
Software is deemed fit for purpose if it meets all of the requirements set out in the analysis stage.
For example, if a program was expected to take in ten test scores and provide an average but instead it displayed the total, it would not be fit for purpose since it does not meet the original requirements.
A solution must be revisited and refined until it meets the original requirements for it to be deemed as fit for purpose.
Efficient use of constructs
At National 5, you need to consider that software uses arrays instead of many variable declarations, uses loops where possible to reduce the amount of code written and whether they have used nested if statements where possible.
Using arrays
At National 5, using arrays instead of multiple variables is one of the most common ways to evaluate a program in terms of efficiency:
mark1 = int(input("Please enter mark number 1")) mark2 = int(input("Please enter mark number 2")) mark3 = int(input("Please enter mark number 3")) mark4 = int(input("Please enter mark number 4"))
This could be made more efficient by using arrays:
marks = [0] * 4 marks[0] = int(input("Please enter mark number 1")) marks[1] = int(input("Please enter mark number 2")) marks[2] = int(input("Please enter mark number 3")) marks[3] = int(input("Please enter mark number 4"))
Fixed loops
A pupils marks are stored as shown below:
marks = [0] * 4 marks[0] = int(input("Please enter mark number 1")) marks[1] = int(input("Please enter mark number 2")) marks[2] = int(input("Please enter mark number 3")) marks[3] = int(input("Please enter mark number 4")) print(marks[0]) print(marks[1]) print(marks[2]) print(marks[3])
This could be made more efficient using loops:
for counter in range(0, 4): marks[counter] = int(input("Please enter mark number" + str(counter + 1))) for counter in range(0, 4): print(marks[counter])
Nested if statements
percentage = int(input("Please enter your percentage")) if percentage >= 70) grade = "A" if percentage >= 60 And percentage < 70: grade = "B" if percentage >= 50 And percentage < 60: grade = "C" if percentage >= 40 And percentage < 50: grade = "D" if percentage < 40: grade = "F"
This can be made more efficient by combining results using else
statements (in terms of
compilation this is the worst option but in terms of best performance it comes equal with the one following):
percentage = int(input("Please enter your percentage")) if percentage >= 70: grade = "A" else if percentage >= 60 And percentage < 70: grade = "B" else if percentage >= 50 And percentage < 60: grade = "C" else if percentage >= 40 And percentage < 50: grade = "D" else: grade = "F"
Or, even better, using elif
blocks (in terms of both compilation and runtime performance,
this is by far the best option):
percentage = int(input("Please enter your percentage")) if percentage >= 70: grade = "A" elif percentage >= 60 And percentage < 70: grade = "B" elif percentage >= 50 And percentage < 60: grade = "C" elif percentage >= 40 And percentage < 50: grade = "D" else: grade = "F"